This post is a complete project that will cover many things; you will learn how to create a web api, how to create a fixture, how to create a unit testing with xUnit and how to implement integration test with wiremock.net.
The project is a c# unit test mock tutorial, we are working on a .NET 5.0 c# web api project, it is a full project where a console app is calling a weather web api, and we create a unit test with xUnit that test the web api. In order to test a web api, we need wiremock. WireMock allow us to mock the behavior of the api, no need to send real remote request and wait response, with wiremock you can pretend to send a request and get a real response.
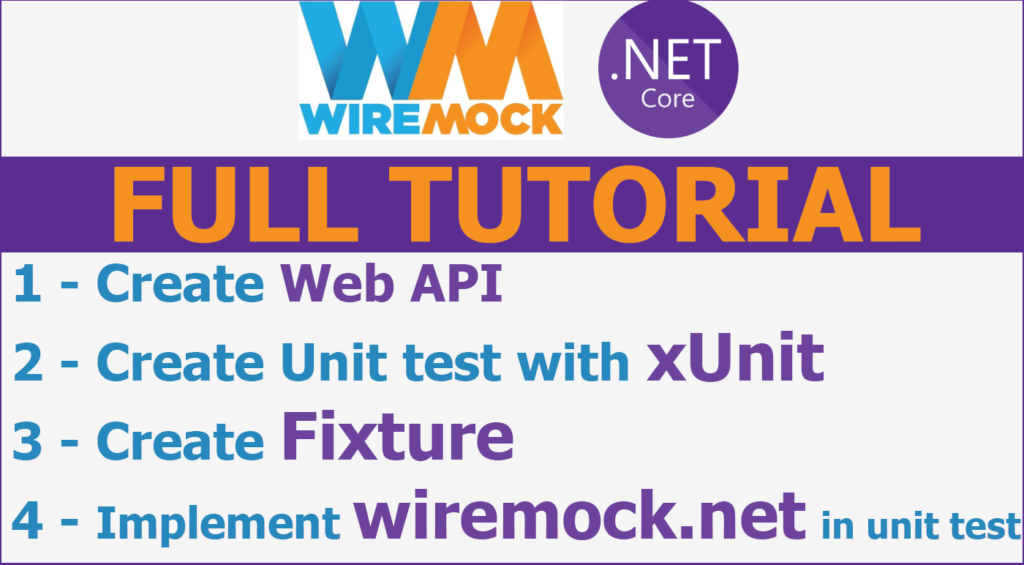
Project structure
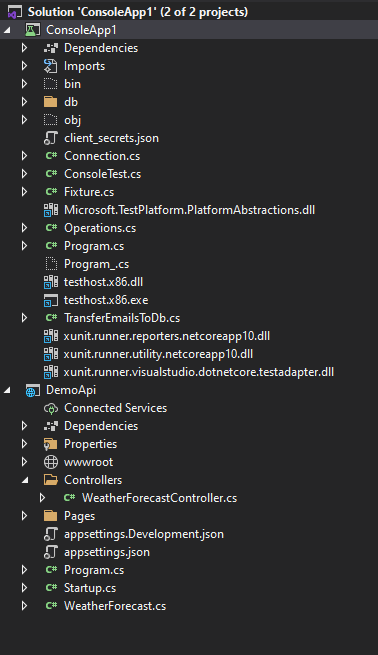
Our solution contains 2 projects; ConsoleApp and DemoApi
The ConsoleApp will contain the unit test (ConsoleTest) which is a xUnit that will execute testing on the DemoApi and a fixture (Fixture.cs) which contains the wiremock.net implementation.
ConsoleTest.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
using Xunit;
namespace ConsoleApp1
{
public class ConsoleTest : IClassFixture<Fixture>
{
Fixture fixture;
public ConsoleTest(Fixture fixture)
{
this.fixture = fixture;
}
[Fact]
public async Task Should_GetWeatherForecastData_When_ServiceAvailable()
{
HttpClientHandler clientHandler = new HttpClientHandler();
clientHandler.ServerCertificateCustomValidationCallback = (sender, cert, chain, sslPolicyErrors) => { return true; };
HttpClient client = new HttpClient(clientHandler);
fixture.WireMockServerRun(44363, "/WeatherForecast", 200,"Any Response Message","Get");
var requestResult = await client.GetAsync("https://localhost:44363/WeatherForecast");
Assert.True(requestResult.StatusCode == HttpStatusCode.OK);
}
}
}
Fixture.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using WireMock.RequestBuilders;
using WireMock.Server;
namespace ConsoleApp1
{
public class Fixture : IDisposable
{
//public DbContext context { get; private set; }
public WireMockServer wireMockServer;
public Fixture()
{
}
public void InitDatabase()
{
//var options = new DbContextOptionsBuilder<YodifemDb>().UseInMemoryDatabase(databaseName: "YodifemDb").Options;
//context = new DbContext(options);
}
public void WireMockServerRun(int port, string givenPath, int statusCode, string Response, string Method)
{
wireMockServer = WireMockServer.Start(port, ssl:true);
if (Method.Equals("Post"))
wireMockServer
.Given(Request.Create().WithPath(givenPath).UsingPost())
.RespondWith(WireMock.ResponseBuilders.Response.Create().WithStatusCode(statusCode)
.WithBody(Response)
);
else if (Method.Equals("Get"))
wireMockServer
.Given(Request.Create().WithPath(givenPath).UsingGet())
.RespondWith(WireMock.ResponseBuilders.Response.Create().WithStatusCode(statusCode)
.WithBody(Response)
);
else
wireMockServer
.Given(Request.Create().WithPath(givenPath))
.RespondWith(WireMock.ResponseBuilders.Response.Create().WithStatusCode(statusCode)
.WithBody(Response)
);
}
public void Dispose()
{
//context.Dispose();
}
}
}
In ConsoleTest, the line
fixture.WireMockServerRun(44363, “/WeatherForecast”, 200,”Any Response Message”,”Get”);
This line will create a fake server on port 44363, and it will allow to send fake request to it. And the response will be HttpOK (200) and “Any Response Message“.
For a complete Demo, you can check this wiremock.net video tutorial on YouTube:
https://youtu.be/zzYEwQinMBE